Web Component Installation & Usage
Vertical Insure provides a simple set of web components for retrieving insurance quotes in your checkout page.
Latest Web Component Version
Including from CDN
We provide a non-bundled and bundled version of our web component module to. We recommend only using the non-bundled approach for testing as the user will need to download every javascript dependency from the CDN as well. You should always reference a specific version as pulling the latest could result in pulling in breaking changes.
<script src="https://unpkg.com/@vertical-insure/web-components@{VERSION}/index.bundle.js"></script>
Installing into a javascript project
You can install the web component into any javascript module using
npm install @vertical-insure/web-components
And depending on the framework you are using, you can simply import the module and you'll be able to use in standard HTML or JSX.
import "@vertical-insure/web-components";
Configuration
All of our web components require a client-id
attribute. This value is part of your API credentials and comes from generating API keys. See Authentication for more details.
Getting the quote information
<script>
const insuranceComponent = document.querySelector('registration-cancellation');
console.log(insuranceComponent.quote);
</script>
Validation for forms
Some components are defined as "required" and will need to be validated. For example, a component with a radio form input would require a selection "yes" or "no"
Every components validation is updated as the component changes and isValid
can be determined based on the change
event defined below.
<script>
const insuranceComponent = document.querySelector('registration-cancellation');
const validation = insuranceComponent.validate();
if (validation.isValid) {
form.submit();
}
</script>
Event Listeners
offer-state-change
The offer-state-change
is a CustomEvent
and will contain the following:
{
detail: {
quote: {...},
selectedState: 'string' // this can be either ACCEPTED, DECLINED, UNKNOWN
}
}
This event is triggered whenever a quote is updated or when a user takes action within the component.
<script>
const insuranceComponent = document.querySelector('registration-cancellation');
insuranceComponent.addEventListener('offer-state-change', function(event) {
if (event.detail.selectedState === "ACCEPTED") {
addInsuranceToCart(event.detail.quote.quote_id, event.detail.quote.premium_amount);
}
});
</script>
Debugging
You can also set the debug
flag on any component to get events logged to the console in your web browser.
<registration-cancellation ... debug="true"></registration-cancellation>
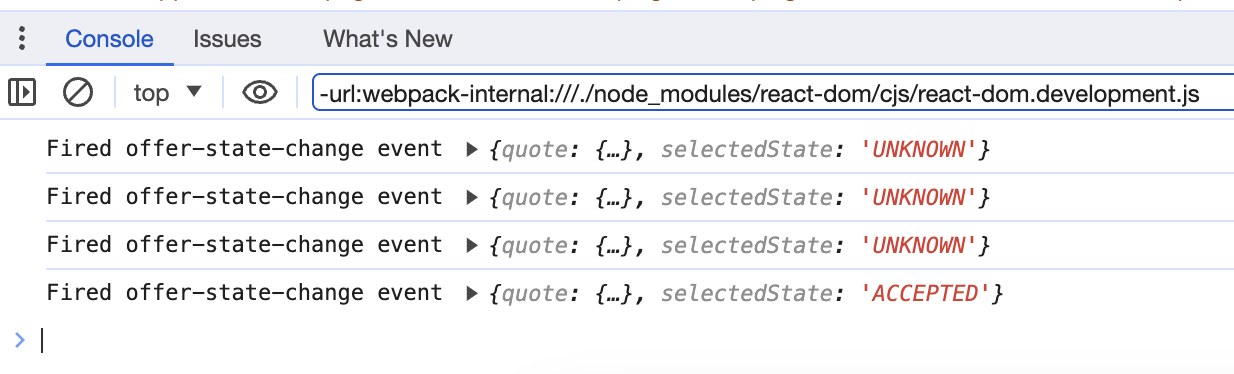